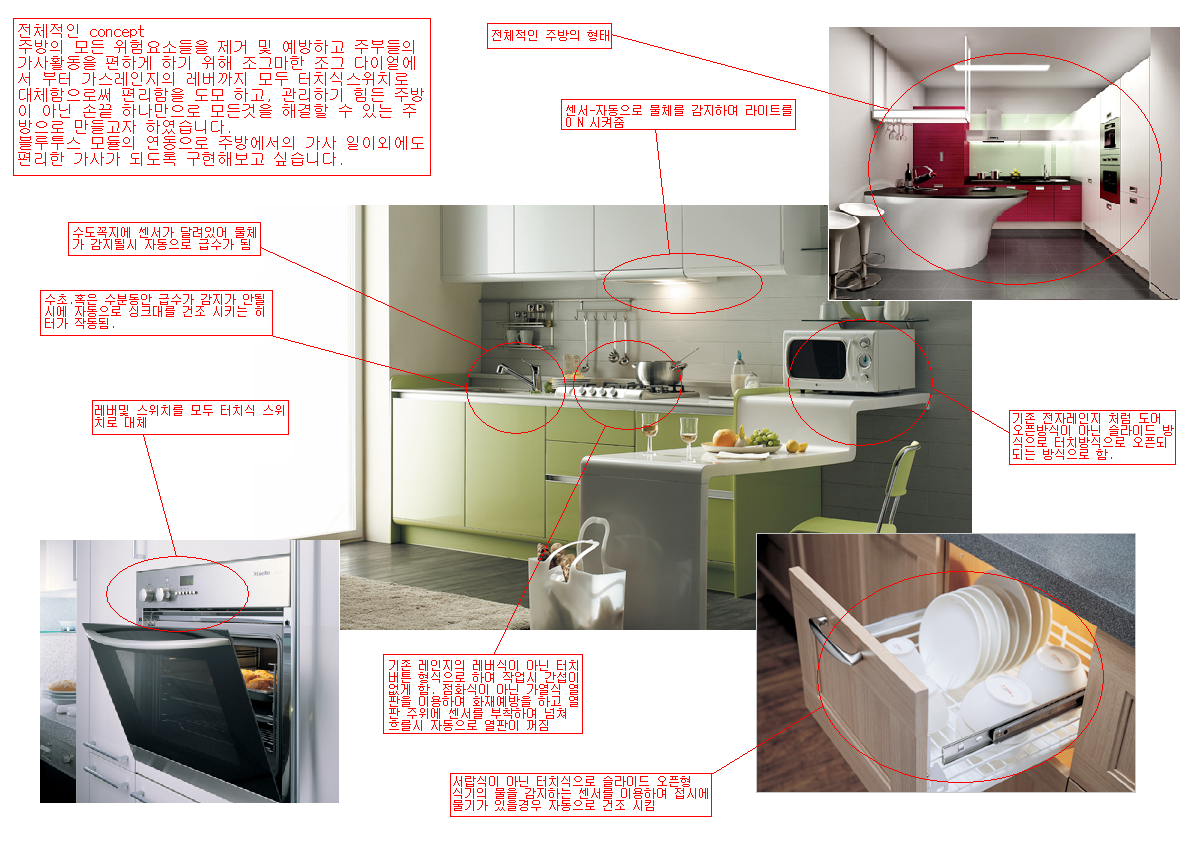
#include<iom128.h>
#include<ina90.h>
unsigned int data=0x00;
#define ENABLE (PORTA_Bit2=1)
#define DISABLE (PORTA_Bit2=0)
void init_PORTA(void)
{
DDRA = 0xFF;
PORTA = 0xFF;
}
void wait_busy_flag(void)
{
unsigned int i;
for(i=0; i<10000; i++);
}
void m_delay(unsigned int m)
{
unsigned int i,j;
for(i=0; i<m; i++)
for(j=0; j<2650; j++);
}
void ddelay(unsigned int p)
{
unsigned int i;
for(i=0; i<p; i++);
}
void instruction_out(unsigned char b)
{
PORTA=b&0xF0;
ENABLE;
DISABLE;
PORTA=(b<<4)&0xF0;
ENABLE;
DISABLE;
m_delay(2);
}
void char_out(unsigned char b)
{
PORTA=(b&0xF0) | 0x01;
ENABLE;
DISABLE;
PORTA=((b<<4)&oxF0) | 0x01;
ENABLE;
DISABLE;
m_delay(2);
}
void string_out(unsigned char b, unsigned char* str)
{
unsigned int i=0;
instruction_out(b);
do{
char_out(str[i]);
}while(str[++i]!='\0');
}
void init_LCD(void)
{
init_PORTA();
m_delay(15);
ddelay(600);
instruction_out(0x28);
m_delay(2);
instruction_out(0x28);
m_delay(2);
instruction_out(0x0C);
instruction_out(0x06);
instruction_out(0x02);
instruction_out(0x01);
instruction_out(0x01);
}
void lcd_decimal(unsigned char b, unsigned int hex)
{
unsigned int h;
instruction_out(b);
h = (hex/1000);
if(h != 0)
char_out(h + 0x30);
else
char_out(0x30);
h= ((hex % 1000) / 100);
if( h!= 0)
char_out(h + 0x30);
else
char_out(0x30);
h = (((hex % 1000) 100) /10);
if( h != 0)
char_out(h + 0x30);
else
char_out(0x30);
h = (((hex % 1000) % 100) % 10);
if(h != 0)
char_out(h + 0x30);
else
char_out(0x30);
}
void main(void)
{
DDRB=0xFF;
PORTB=0xFF;
DDRD_Bit0=0;
init_LCD();
EIMSK |= 0x01;
EICRA |= 0x03;
EIFR |= 0x01;
instruction_out(0x01);
string_out(0x80,"JSLEE");
__enable_interrupt()
while(1)
lcd_decimal(0xC2,data);
}
#pragma vector=INT0_vect
__interrupt void INT0_interrupt(void)
{
__disable_interrupt();
data++;
__enable_interrupt();
}